test_data_generation¶
Module: tests.utils.data.test_data_generation
¶
Data generation facilities to test algorithms or node chains e.g. in unittests
Inheritance diagram for pySPACE.tests.utils.data.test_data_generation
:
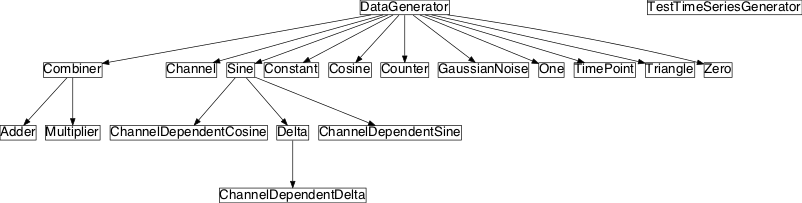
Class Summary¶
DataGenerator ([sampling_frequency]) |
Abstract base class for data generation for different test data patterns |
Zero (\*args, \*\*kwargs) |
Helper function for data generation that simply returns zero |
One (\*args, \*\*kwargs) |
Helper function for data generation that simply returns one |
Constant (value, \*args, \*\*kwargs) |
Helper function for data generation that simply returns one |
Counter ([start]) |
Counts the number of calls and returns the value |
Channel (num_channels, num_time_pts, \*args, ...) |
Generated the number of the actual channel |
TimePoint (num_channels, num_time_pts, \*args, ...) |
Generated the index of the actual time point |
Triangle (width, height, \*args, \*\*kwargs) |
Generates a triangle with a given width and height |
GaussianNoise ([mean, std, seed]) |
Generates normal distributed noise |
Sine ([phase, frequency, amplitude, ...]) |
Generates a sine wave |
ChannelDependentSine (\*args, \*\*kwargs) |
Generates a sine wave with channel scaled frequency |
Cosine ([phase, frequency, amplitude, ...]) |
Generates a cosine wave |
ChannelDependentCosine (\*args, \*\*kwargs) |
Generates a cosine wave with channel scaled frequency |
Delta ([k]) |
Generates a delta impulse, i.e. |
ChannelDependentDelta (\*args, \*\*kwargs) |
Generates a sine wave with channel scaled frequency |
Combiner ([generator_list]) |
Combines several generators |
Adder ([generator_list]) |
Combines several signal by adding them together |
Multiplier ([generator_list]) |
Combines several signal by adding them together |
TestTimeSeriesGenerator |
Helper class to generate time series objects e.g. |
Classes¶
DataGenerator
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
DataGenerator
(sampling_frequency=1.0, *args, **kwargs)[source]¶ Bases:
object
Abstract base class for data generation for different test data patterns
To implement an arbitrary data generation class, subclass from this class and override the method generate() . This can be sine waves, different types of noise, etc.
Class Components Summary
__abstractmethods__
__call__
()Helper function that returns, how often it was called _abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()get_sampling_frequency
()next_channel
()Goes to the next channel sampling_frequency
set_sampling_frequency
(sampling_frequency)-
sampling_frequency
¶
-
__metaclass__
¶ alias of
ABCMeta
-
__abstractmethods__
= frozenset(['generate'])¶
-
__weakref__
¶ list of weak references to the object (if defined)
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Zero
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Zero
(*args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Helper function for data generation that simply returns zero
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
One
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
One
(*args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Helper function for data generation that simply returns one
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Constant
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Constant
(value, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Helper function for data generation that simply returns one
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Counter
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Counter
(start=0, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Counts the number of calls and returns the value
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Channel
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Channel
(num_channels, num_time_pts, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Generated the number of the actual channel
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
TimePoint
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
TimePoint
(num_channels, num_time_pts, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Generated the index of the actual time point
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Triangle
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Triangle
(width, height, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Generates a triangle with a given width and height
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
GaussianNoise
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
GaussianNoise
(mean=0.0, std=1.0, seed=None, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Generates normal distributed noise
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Sine
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Sine
(phase=0.0, frequency=1.0, amplitude=1.0, sampling_frequency=1.0, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Generates a sine wave
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
ChannelDependentSine
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
ChannelDependentSine
(*args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.Sine
Generates a sine wave with channel scaled frequency
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
next_channel
()Goes to the next channel -
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Cosine
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Cosine
(phase=0.0, frequency=1.0, amplitude=1.0, sampling_frequency=1.0, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Generates a cosine wave
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
ChannelDependentCosine
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
ChannelDependentCosine
(*args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.Sine
Generates a cosine wave with channel scaled frequency
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
next_channel
()Goes to the next channel -
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Delta
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Delta
(k=0, *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.Sine
Generates a delta impulse, i.e. 1 if t==-k, 0 else
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
ChannelDependentDelta
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
ChannelDependentDelta
(*args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.Delta
Generates a sine wave with channel scaled frequency
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()next_channel
()Goes to the next channel -
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Combiner
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Combiner
(generator_list=[], *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.DataGenerator
Combines several generators
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
add_generator
(generator)get_sampling_frequency
()sampling_frequency
set_sampling_frequency
(sampling_frequency)-
sampling_frequency
¶
-
__abstractmethods__
= frozenset(['generate'])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Adder
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Adder
(generator_list=[], *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.Combiner
Combines several signal by adding them together
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
Multiplier
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
Multiplier
(generator_list=[], *args, **kwargs)[source]¶ Bases:
pySPACE.tests.utils.data.test_data_generation.Combiner
Combines several signal by adding them together
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
generate
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
TestTimeSeriesGenerator
¶
-
class
pySPACE.tests.utils.data.test_data_generation.
TestTimeSeriesGenerator
[source]¶ Bases:
object
Helper class to generate time series objects e.g. by DataGenerator classes
Class Components Summary
add_to_test_data
(time_series, function)Function to add an additional signal generated by function to an existing time series add_to_test_data_single_channel
(time_series, ...)generate_normalized_test_data
(channels, ...)A method which generates a normalized (mu = 0, sigma =1) signal for testing, with generate_test_data
([channels, time_points, ...])A method which generates a signal for testing, with the specified number of “channels” which are all generated using the given function. generate_test_data_simple
(channels, ...[, ...])A method which generates a signal by using function for testing, with the specified number of “channels” which are all generated using the given function. init
(\*\*kwargs)-
generate_test_data
(channels=1, time_points=100, function=<pySPACE.tests.utils.data.test_data_generation.Sine object>, sampling_frequency=1000, channel_order=True, channel_names=None, dtype=<type 'float'>)[source]¶ A method which generates a signal for testing, with the specified number of “channels” which are all generated using the given function.
Keyword arguments
channels: number of channels time_points: number of time points function: the function used for sample generation sampling_frequency: the frequency which is used for sampling, e.g. the signal corresponds to a time frame of time_points/sampling frequency channel_names: the names of the channels (alternative to the channels parameter, if not None, it also specifies the number of channels) channel_order: the channel values are computed first, use False for first computation of the row values dtype: data type of the array
-
generate_test_data_simple
(channels, time_points, function, sampling_frequency, initial_phase=0.0)[source]¶ A method which generates a signal by using function for testing, with the specified number of “channels” which are all generated using the given function.
Keyword arguments
channels: number of channels time_points: number of time points function: the function used for sample generation sampling_frequency: the frequency which is used for sampling, e.g. the signal corresponds to a time frame of time_points/sampling frequency
-
add_to_test_data
(time_series, function)[source]¶ Function to add an additional signal generated by function to an existing time series
Keyword arguments
timeSeries: the time series object function: function to generate signal
-
generate_normalized_test_data
(channels, time_points, function, sampling_frequency, initial_phase=0.0)[source]¶ A method which generates a normalized (mu = 0, sigma =1) signal for testing, with the specified number of “channels” which are all generated using the given function
-
__weakref__
¶ list of weak references to the object (if defined)
-