progressbar¶
Module: tools.progressbar
¶
Text progressbar library for Python
This library provides a text mode progressbar. This is typically used to display the progress of a long running operation, providing a visual clue that processing is underway.
The ProgressBar class manages the progress, and the format of the line is given by a number of widgets. A widget is an object that may display differently depending on the state of the progress. There are three types of widget:
- a string, which always shows itself;
- a ProgressBarWidget, which may return a different value every time it’s update method is called; and
- a ProgressBarWidgetHFill, which is like ProgressBarWidget, except it expands to fill the remaining width of the line.
The progressbar module is very easy to use, yet very powerful. And automatically supports features like auto-resizing when available.
Changes in comparison to original code:
- added day display in ETA class
- small style improvements in documentation
- coding style adaptions
Original LGPL 2.1+ license:
Copyright (c) 2005 Nilton Volpato
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
Inheritance diagram for pySPACE.tools.progressbar
:
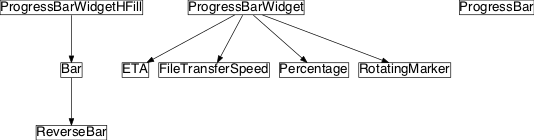
Class Summary¶
ProgressBarWidget |
Element of ProgressBar formatting. |
ProgressBarWidgetHFill |
Variable width element of ProgressBar formatting |
ETA |
Widget for the Estimated Time of Arrival |
FileTransferSpeed () |
Widget for showing the transfer speed (useful for file transfers) |
RotatingMarker ([markers]) |
A rotating marker for filling the bar of progress |
Percentage |
Just the percentage done |
Bar ([marker, left, right]) |
The bar of progress. |
ReverseBar ([marker, left, right]) |
The reverse bar of progress, or bar of regress |
ProgressBar ([maxval, widgets, term_width, fd]) |
Updates and print the progress bar |
Classes¶
ProgressBarWidget
¶
-
class
pySPACE.tools.progressbar.
ProgressBarWidget
[source]¶ Bases:
object
Element of ProgressBar formatting.
The ProgressBar object will call it’s update value when an update is needed. It’s size may change between call, but the results will not be good if the size changes drastically and repeatedly.
Class Components Summary
update
(pbar)Returns the string representing the widget -
update
(pbar)[source]¶ Returns the string representing the widget
The parameter pbar is a reference to the calling ProgressBar, where one can access attributes of the class for knowing how the update must be made.
At least this function must be overridden.
-
__weakref__
¶ list of weak references to the object (if defined)
-
ProgressBarWidgetHFill
¶
-
class
pySPACE.tools.progressbar.
ProgressBarWidgetHFill
[source]¶ Bases:
object
Variable width element of ProgressBar formatting
The ProgressBar object will call it’s update value, informing the width this object must the made. This is like TeX
\hfill
, it will expand to fill the line. You can use more than one in the same line, and they will all have the same width, and together will fill the line.Class Components Summary
update
(pbar, width)Returns the string representing the widget -
update
(pbar, width)[source]¶ Returns the string representing the widget
The parameter pbar is a reference to the calling ProgressBar, where one can access attributes of the class for knowing how the update must be made. The parameter width is the total horizontal width the widget must have.
At least this function must be overridden.
-
__weakref__
¶ list of weak references to the object (if defined)
-
ETA
¶
-
class
pySPACE.tools.progressbar.
ETA
[source]¶ Bases:
pySPACE.tools.progressbar.ProgressBarWidget
Widget for the Estimated Time of Arrival
Class Components Summary
format_time
(seconds)update
(pbar)
Bar
¶
-
class
pySPACE.tools.progressbar.
Bar
(marker='#', left='|', right='|')[source]¶ Bases:
pySPACE.tools.progressbar.ProgressBarWidgetHFill
The bar of progress. It will stretch to fill the line
Class Components Summary
_format_marker
(pbar)update
(pbar, width)
ProgressBar
¶
-
class
pySPACE.tools.progressbar.
ProgressBar
(maxval=100, widgets=[<pySPACE.tools.progressbar.Percentage object>, ' ', <pySPACE.tools.progressbar.Bar object>], term_width=None, fd=None)[source]¶ Bases:
object
Updates and print the progress bar
The term_width parameter may be an integer. Or None, in which case it will try to guess it, if it fails it will default to 80 columns.
The simple use is like this:
>>> pbar = ProgressBar().start() >>> for i in xrange(100): ... # do something ... pbar.update(i+1) ... >>> pbar.finish()
But anything you want to do is possible (well, almost anything). You can supply different widgets of any type in any order. And you can even write your own widgets! There are many widgets already shipped and you should experiment with them.
When implementing a widget update method you may access any attribute or function of the ProgressBar object calling the widget’s update method. The most important attributes you would like to access are:
- currval: current value of the progress, 0 <= currval <= maxval
- maxval: maximum (and final) value of the progress
- finished: True if the bar is have finished (reached 100%), False o/w
- start_time: first time update() method of ProgressBar was called
- seconds_elapsed: seconds elapsed since start_time
- percentage(): percentage of the progress (this is a method)
Class Components Summary
_format_line
()_format_widgets
()_need_update
()finish
()Used to tell the progress is finished handle_resize
(signum, frame)percentage
()Returns the percentage of the progress start
()Start measuring time, and prints the bar at 0%. update
(value)Updates the progress bar to a new value -
__init__
(maxval=100, widgets=[<pySPACE.tools.progressbar.Percentage object>, ' ', <pySPACE.tools.progressbar.Bar object>], term_width=None, fd=None)[source]¶
-
start
()[source]¶ Start measuring time, and prints the bar at 0%.
It returns self so you can use it like this:
>>> pbar = ProgressBar().start() >>> for i in xrange(100): ... # do something ... pbar.update(i+1) ... >>> pbar.finish()
-
__weakref__
¶ list of weak references to the object (if defined)