external¶
Module: missions.nodes.classification.svm_variants.external
¶
Wrapper around external SVM variant implementations like LibSVM or LIBLINEAR
Inheritance diagram for pySPACE.missions.nodes.classification.svm_variants.external
:
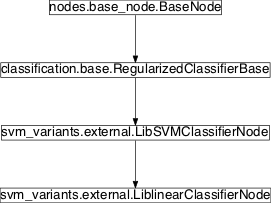
Class Summary¶
LibSVMClassifierNode ([svm_type, ...]) |
Classify like a Standard SVM with the LibSVM settings. |
LiblinearClassifierNode ([tolerance, ...]) |
Code Integration of external linear SVM classifier program |
Classes¶
LibSVMClassifierNode
¶
-
class
pySPACE.missions.nodes.classification.svm_variants.external.
LibSVMClassifierNode
(svm_type='C-SVC', max_iterations=0, str_label_function=None, complexities_path=None, **kwargs)[source]¶ Bases:
pySPACE.missions.nodes.classification.base.RegularizedClassifierBase
Classify like a Standard SVM with the LibSVM settings.
This node is a wrapper around the current libsvm implementation of a SVM.
http://www.csie.ntu.edu.tw/~cjlin/libsvm/oldfiles/
Parameters
Some general parameters are only documented in the
RegularizedClassifierBase
.svm_type: Defines the used SVM type. One of the following Strings: ‘C-SVC’, ‘one-class SVM’, ‘epsilon-SVR’, ‘nu-SVR’. The last two types are for regression, the first for classification.
Warning
For using “one-class SVM” better use the
LibsvmOneClassNode
.(optional, default: ‘C-SVC’)
complexity: Defines parameter for ‘C-SVC’, ‘epsilon-SVR’ and ‘nu-SVR’. Complexity sets the weighting of punishment for misclassification in comparison to generalizing classification from the data. Equals parameter /cost/ or /C/ in libsvm-package. Value in the range from 0 to infinity.
(optional, default: 1)
str_label_function: A String representing a Python eval()-able function, that transforms the labels (list). It makes only sense for numeric labels. E.g. “lambda liste: [exp(-0.0001*elem**2) for elem in liste]”.
(optional, default: None)
debug: If debug is True one gets additional output concerning the classification.
Note
This makes only sense for the ‘LINEAR’-kernel_type.
(optional, default: False)
store: Parameter of super-class. If store is True, the classification vector is stored as a feature vector.
Note
This makes only sense for the ‘LINEAR’-kernel_type.
(optional, default: False)
max_iterations: Restricts the solver inside the LibSVM to maximal use N iterations, where N is the product of max_iterations and the number of samples used to train the classifier. If omitted or set to zero the solver takes as much iterations it needs to calculate the model.
Note
This number has to be an integer and is very important if you expect the classifier not to converge.
Note
To use this feature you will need the modified libsvm of the external folder in a compiled version. Furthermore you should make sure, that this version is imported, e.g. by adding the path at the beginning of the configuration file paths.
(optional, default: 0)
complexities_path: If a complexities_path is given, the complexity is read from a YAML file. This file has a dict with channel numbers as keys and the corresponding complexity as value. Also, a ‘features_per_channel’ dict entry can be set to calculate channel number based on the number of features. If no ‘features_per_channel’ is given, a factor of 1 is assumed. This can be used to specify the number of features in the file, instead of the number of sensor channels. A minimal example for the file content could be:
{32: 0.081, 62: 0.019, features_per_channel: 6}.
‘complexities_path’ will overwrite ‘complexity’.
(optional, default: 0)
Exemplary Call
- node : LibSVM_Classifier parameters : svm_type : "C-SVC" complexity : 1 kernel_type : "LINEAR" class_labels : ['Standard', 'Target'] weight : [1,3] debug : True store : True max_iterations : 100
Input: FeatureVector
Output: PredictionVector
Author: Jan Hendrik Metzen (jhm@informatik.uni-bremen.de) & Mario Krell (Mario.krell@dfki.de)
Created: 2009/07/02
Revised: 2010/04/09
Last change: 2011/05/06 Mario Krell old version deleted
POSSIBLE NODE NAMES: - LibSVM_Classifier
- LibSVMClassifier
- 2SVM
- LibSVMClassifierNode
POSSIBLE INPUT TYPES: - FeatureVector
Class Components Summary
__hyperparameters
_complete_training
([debug])Iterate over the complete data to get the initial model _execute
(x)Executes the classifier on the given data vector x. _stop_training
([debug])Finish the training, i.e. add_new_sample
(data[, class_label, default])Add a new sample to the training set. calculate_classification_vector
(model)Calculate classification vector w and the offset b calculate_slack_variables
(model)This method calculates from the given SVM model the related slack variables for classification. input_types
load_model
(filename)remove_no_border_points
(retraining_required)Discard method to remove all samples from the training set that are not in the border of their class. remove_samples
(idxs)Remove the samples at the given indices from the training set. save_model
(filename)visualize
()Show the training samples, SVS and the current decision function -
__init__
(svm_type='C-SVC', max_iterations=0, str_label_function=None, complexities_path=None, **kwargs)[source]¶
-
_execute
(x)[source]¶ Executes the classifier on the given data vector x. prediction value = <w,data>+b in the linear case.
-
calculate_slack_variables
(model)[source]¶ This method calculates from the given SVM model the related slack variables for classification.
-
remove_no_border_points
(retraining_required)[source]¶ Discard method to remove all samples from the training set that are not in the border of their class.
The border is determined by a minimum distance from the center of the class and a maximum distance.
Parameters: retraining_required – flag if retraining is requiered (the new point is a potential SV or a removed one was a sv)
-
add_new_sample
(data, class_label=None, default=False)[source]¶ Add a new sample to the training set.
Parameters: - data (list of float) – A new sample for the training set.
- class_label (str) – The label of the new sample.
- default – Specifies if the sample is added to the current training set or to a future training set
- default – bool
-
remove_samples
(idxs)[source]¶ Remove the samples at the given indices from the training set.
Param: idxs: Indices of the samples to remove. Type: idxs: list of int Return type: bool - True if a support vector was removed.
-
__hyperparameters
= set([ChoiceParameter<kernel_type>, NormalParameter<ratio>, NoOptimizationParameter<input_dim>, NoOptimizationParameter<dtype>, NoOptimizationParameter<output_dim>, QNormalParameter<offset>, NoOptimizationParameter<use_list>, UniformParameter<nu>, NoOptimizationParameter<retrain>, LogUniformParameter<complexity>, NoOptimizationParameter<keep_vectors>, NoOptimizationParameter<kwargs_warning>, LogNormalParameter<epsilon>, NoOptimizationParameter<debug>, QUniformParameter<max_time>, LogNormalParameter<tolerance>, NoOptimizationParameter<regression>, NoOptimizationParameter<store>])¶
-
input_types
= ['FeatureVector']¶
LiblinearClassifierNode
¶
-
class
pySPACE.missions.nodes.classification.svm_variants.external.
LiblinearClassifierNode
(tolerance=0.001, svm_type=3, offset=True, **kwargs)[source]¶ Bases:
pySPACE.missions.nodes.classification.svm_variants.external.LibSVMClassifierNode
Code Integration of external linear SVM classifier program
http://www.csie.ntu.edu.tw/~cjlin/liblinear/ LIBLINEAR was implemented by the LIBSVM programmers.
It is important to mention, that here (partially) the same modified SVM model is used as in the SOR variant. (
pySPACE.missions.nodes.classification.svm_variants.SOR
)Parameters
Some general parameters are only documented in the
RegularizedClassifierBase
.svm_type: 0: L2-regularized logistic regression (primal) 1: L2-regularized L2-loss support vector classification (dual) 2: L2-regularized L2-loss support vector classification (primal) 3: L2-regularized L1-loss support vector classification (dual) 4: multi-class support vector classification by Crammer and Singer 5: L1-regularized L2-loss support vector classification 6: L1-regularized logistic regression 7: L2-regularized logistic regression (dual) Type 3 is the standard SVM with b used in the target function as component of w (offset = True) or b set to zero.
(optional, default:3)
tolerance: Tolerance of termination criterion, same default as in libsvm.
(optional, default: 0.001)
offset: If True, x is internally replaced by (x,1) to get an artificial offset b. Probably in this case b is regularized. Otherwise the offset b in the classifier function (w^Tx+b) is set to zero.
(optional, default: True)
store: Parameter of super-class. If store is True, the classification vector is stored as a feature vector.
(optional, default: False)
Exemplary Call
- node : lSVM parameters : class_labels : ["Target", "Standard"]
Author: Mario Michael Krell (mario.krell@dfki.de)
Created: 2012/01/19
POSSIBLE NODE NAMES: - LiblinearClassifierNode
- lSVM
- LiblinearClassifier
POSSIBLE INPUT TYPES: - FeatureVector
Class Components Summary
__hyperparameters
_complete_training
([debug])Forward data to external training and extract classifier information _stop_training
([debug])Finish the training, i.e. _train
(data, class_label)Trains the classifier on the given data calculate_classification_vector
(model)This method calculates from the given SVM model the related classification vector w and the offset b. -
_train
(data, class_label)[source]¶ Trains the classifier on the given data
It is assumed that the class_label parameter contains information about the true class the data belongs to
-
_complete_training
(debug=False)[source]¶ Forward data to external training and extract classifier information
-
__hyperparameters
= set([ChoiceParameter<kernel_type>, NormalParameter<ratio>, NoOptimizationParameter<input_dim>, UniformParameter<nu>, NoOptimizationParameter<dtype>, NoOptimizationParameter<output_dim>, QNormalParameter<offset>, NoOptimizationParameter<use_list>, ChoiceParameter<svm_type>, NoOptimizationParameter<retrain>, LogUniformParameter<complexity>, NoOptimizationParameter<keep_vectors>, NoOptimizationParameter<kwargs_warning>, LogNormalParameter<epsilon>, NoOptimizationParameter<debug>, QUniformParameter<max_time>, LogNormalParameter<tolerance>, NoOptimizationParameter<regression>, NoOptimizationParameter<store>])¶