decorators¶
Module: missions.nodes.decorators
¶
Define parameter distributions for pySPACE nodes.
Inheritance diagram for pySPACE.missions.nodes.decorators
:
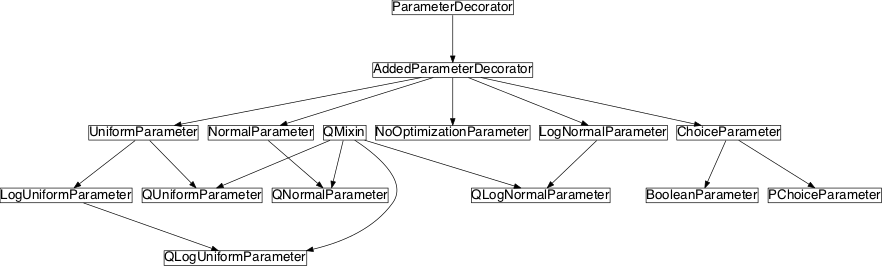
Class Summary¶
ParameterDecorator (parameter_name) |
Abstract base class for creating parameter decorators to declare the as optimization parameters. |
AddedParameterDecorator (parameter_name) |
This mixin adds an execute method to classes that inherit from it. |
QMixin (q) |
This mixin adds a regulation parameter q to bind a distribution to discrete values. |
ChoiceParameter (parameter_name, choices) |
Defines a parameter as to be chosen from the given set of options. |
BooleanParameter (parameter_name) |
Defines a parameter as a being a boolean parameter. |
PChoiceParameter (parameter_name, choices) |
Defines a parameter as a probability choice. |
NormalParameter (parameter_name, mu, sigma) |
Defines a parameter as being normal distributed. |
UniformParameter (parameter_name, min_value, ...) |
Defines a parameter as being uniform distributed. |
QNormalParameter (parameter_name, mu, sigma, q) |
Defines a parameter as being normal distributed but to only take discrete values. |
QUniformParameter (parameter_name, min_value, ...) |
Defines a parameter as being uniform distributed but to take only discrete values. |
LogNormalParameter (parameter_name, shape, scale) |
Defines a parameter as being drawn from the exponential of a normal distribution. |
LogUniformParameter (parameter_name, ...) |
Defines a parameter as being drawn from the exponential of a uniform distribution. |
QLogNormalParameter (parameter_name, shape, ...) |
Defines a parameter as being drawn from the exponential of a normal distribution. |
QLogUniformParameter (parameter_name, ...) |
Defines a parameter as being drawn from the exponential of a uniform distribution and being bound to discrete values only. |
NoOptimizationParameter (parameter_name) |
Defines a previously defined parameter as not being an optimization parameter at all. |
Classes¶
ParameterDecorator
¶
-
class
pySPACE.missions.nodes.decorators.
ParameterDecorator
(parameter_name)[source]¶ Bases:
object
Abstract base class for creating parameter decorators to declare the as optimization parameters.
BE CAREFUL WHEN IMPLEMENTING NEW DECORATORS. THEY MUST BE WRAPPED AND SUPPORTED BY __ALL__ OPTIMIZATION ALGORITHMS.
Class Components Summary
__abstractmethods__
__call__
(class_)__eq__
(other)__hash__
()__ne__
(other)__repr__
()__str__
()_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
execute
(class_, parameters)Execute the decorator plot
()Plot the given parameter distribution -
__metaclass__
¶ alias of
ABCMeta
-
__init__
(parameter_name)[source]¶ Create a new optimization parameter with parameter_name as name
The names of parameters have to be unique, as they get identified by the name.
Parameters: parameter_name (str) – The name of the parameter to create.
-
execute
(class_, parameters)[source]¶ Execute the decorator
This method will be called during creation of the class object and will update the given set of hyperparameters according to the implementation of the subclass.
Parameters: - class (type) – The class object this parameter decorates
- parameters (set(ParameterDecorator)) – The set of parameters to append to or delete from
-
plot
()[source]¶ Plot the given parameter distribution
This method is used to plot the specified distribution of this decorator. For plotting either (if installed) the matplotlib.pyplot can be used and return a figure, or (if not installed) a string specifying the distribution can be returned.
Returns: The figure where this distribution is plotted or a string specifying the distribution Return type: matplotlib.figure.Figure | str
-
__abstractmethods__
= frozenset(['plot', 'execute'])¶
-
__weakref__
¶ list of weak references to the object (if defined)
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
AddedParameterDecorator
¶
-
class
pySPACE.missions.nodes.decorators.
AddedParameterDecorator
(parameter_name)[source]¶ Bases:
pySPACE.missions.nodes.decorators.ParameterDecorator
This mixin adds an execute method to classes that inherit from it.
This mixin will check if the given parameter is already defined and if so removes the old definition and adds the new one.
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
execute
(class_, parameters)-
__metaclass__
¶ alias of
ABCMeta
-
__abstractmethods__
= frozenset(['plot'])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
QMixin
¶
-
class
pySPACE.missions.nodes.decorators.
QMixin
(q)[source]¶ Bases:
object
This mixin adds a regulation parameter q to bind a distribution to discrete values.
Class Components Summary
calc_probability
(value, pdf_func)q
round_to_q
(value)Round a value to the next multiple of q. -
q
¶
-
round_to_q
(value)[source]¶ Round a value to the next multiple of q.
This is required for the plotting only.
Parameters: value (float) – The value to round
-
__weakref__
¶ list of weak references to the object (if defined)
-
ChoiceParameter
¶
-
class
pySPACE.missions.nodes.decorators.
ChoiceParameter
(parameter_name, choices)[source]¶ Bases:
pySPACE.missions.nodes.decorators.AddedParameterDecorator
Defines a parameter as to be chosen from the given set of options.
This parameter will be then chosen from this set during the optimization.
..code-block:: python
>>> @ChoiceParameter("test", choices=["A", "B", "C"] ... class A(object): ... pass
Class Components Summary
__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
choices
plot
()-
__init__
(parameter_name, choices)[source]¶ Create a new choice parameter with parameter_name as name and choices as the possible values.
Parameters: - parameter_name (str) – The name of the parameter to create.
- choices (str | List[str]) – The possible values for this parameter.
-
choices
¶
-
__abstractmethods__
= frozenset([])¶
-
__slotnames__
= []¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
BooleanParameter
¶
-
class
pySPACE.missions.nodes.decorators.
BooleanParameter
(parameter_name)[source]¶ Bases:
pySPACE.missions.nodes.decorators.ChoiceParameter
Defines a parameter as a being a boolean parameter.
This parameter will either be “true” or “false” during the optimization.
..code-block:: python
>>> @BooleanParameter("test") ... class A(object): ... pass
Class Components Summary
__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
-
__init__
(parameter_name)[source]¶ Creates a new boolean parameter with parameter_name as name.
Parameters: parameter_name (str) – The name of the parameter to create.
-
__abstractmethods__
= frozenset([])¶
-
__slotnames__
= []¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
PChoiceParameter
¶
-
class
pySPACE.missions.nodes.decorators.
PChoiceParameter
(parameter_name, choices)[source]¶ Bases:
pySPACE.missions.nodes.decorators.ChoiceParameter
Defines a parameter as a probability choice.
This parameter will sample each of the given choices with the according probability.
..code-block:: python
>>> @PChoiceParameter("test", choices={"A": 0.5, "B": 0.25, "C": 0.25}) ... class A(object): ... pass
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
-
__init__
(parameter_name, choices)[source]¶ Create a new probability choice parameter with parameter_name as name and choices as the possible values.
Each choice must be a tuple containing first the probability in range from 0 to 1 for that choice and the value to choose as a second argument. The probabilities of all choices need to sum up to 1.
Parameters: - parameter_name (str) – The name of the parameter to create.
- choices (dict[object, float]) – A dictionary of tuples containing the value of each choice as keys and the corresponding probabilities as values.
-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
NormalParameter
¶
-
class
pySPACE.missions.nodes.decorators.
NormalParameter
(parameter_name, mu, sigma)[source]¶ Bases:
pySPACE.missions.nodes.decorators.AddedParameterDecorator
Defines a parameter as being normal distributed.
A normal distributed parameter will be sampled from the defined distribution by the mean and standard deviation.
This parameter will be sampled from a function like:
normal(mu, sigma)This parameter is unbound.
>>> @NormalParameter("test", mu=0, sigma=1) ... class A(object): ... pass
Class Components Summary
Normal
(mu, sigma)__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
mu
plot
()sigma
-
class
Normal
(mu, sigma)[source]¶ Bases:
object
-
__weakref__
¶ list of weak references to the object (if defined)
-
-
NormalParameter.
__init__
(parameter_name, mu, sigma)[source]¶ Create a new normal distributed parameter with parameter_name as name.
The mean mu and standard deviation sigma are defining the distribution this parameter will be sampled from.
Parameters: - parameter_name (str) – The name of the parameter to create.
- mu (float) – The mean value of the distribution for this parameter
- sigma (float) – The standard deviation of the distribution for this parameter
-
NormalParameter.
mu
¶
-
NormalParameter.
sigma
¶
-
NormalParameter.
__abstractmethods__
= frozenset([])¶
-
NormalParameter.
__slotnames__
= []¶
-
NormalParameter.
_abc_cache
= <_weakrefset.WeakSet object>¶
-
NormalParameter.
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
NormalParameter.
_abc_negative_cache_version
= 33¶
-
NormalParameter.
_abc_registry
= <_weakrefset.WeakSet object>¶
-
class
UniformParameter
¶
-
class
pySPACE.missions.nodes.decorators.
UniformParameter
(parameter_name, min_value, max_value)[source]¶ Bases:
pySPACE.missions.nodes.decorators.AddedParameterDecorator
Defines a parameter as being uniform distributed.
A uniform distributed parameter will be sampled equally distributed between a given minimum and maximum value.
The value of this parameter will be sampled from a function like:
uniform(min, max)
This parameter is bound to [min, max].
>>> @UniformParameter("test", min_value=1, max_value=10) ... class A(object): ... pass
Class Components Summary
Uniform
(a, b)__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
max
min
plot
()-
class
Uniform
(a, b)[source]¶ Bases:
object
-
__weakref__
¶ list of weak references to the object (if defined)
-
-
UniformParameter.
__init__
(parameter_name, min_value, max_value)[source]¶ Create a new uniform distributed parameter with parameter_name as name.
The min_value and max_value define the borders for this distribution in between which the value will be sampled.
Parameters: - min_value (float) – The minimum value of the parameter
- max_value (float) – The maximum value of the parameter
-
UniformParameter.
min
¶
-
UniformParameter.
max
¶
-
UniformParameter.
__abstractmethods__
= frozenset([])¶
-
UniformParameter.
__slotnames__
= []¶
-
UniformParameter.
_abc_cache
= <_weakrefset.WeakSet object>¶
-
UniformParameter.
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
UniformParameter.
_abc_negative_cache_version
= 33¶
-
UniformParameter.
_abc_registry
= <_weakrefset.WeakSet object>¶
-
class
QNormalParameter
¶
-
class
pySPACE.missions.nodes.decorators.
QNormalParameter
(parameter_name, mu, sigma, q)[source]¶ Bases:
pySPACE.missions.nodes.decorators.NormalParameter
,pySPACE.missions.nodes.decorators.QMixin
Defines a parameter as being normal distributed but to only take discrete values.
A q-normal distributed parameter will be sampled from the distribution defined by a mean and a standard deviation but it will be bound to discrete values regularized by a regulation parameter. Therefore the value will be sampled from a function like this:
round(normal(mu, sigma) / q) * qThis parameter is unbound.
>>> @QNormalParameter("test", mu=0, sigma=1, q=0.5) ... class A(object): ... pass
Class Components Summary
__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
plot
()-
__init__
(parameter_name, mu, sigma, q)[source]¶ Creates a new q-normal distributed parameter with parameter_name as name.
The mean mu and standard deviation sigma are defining the distribution this parameter will be sampled from. But it will be bound to discrete values by the regulation parameter q.
Parameters: - parameter_name (str) – The name of the parameter to create.
- mu (float) – The mean value of the distribution for the parameter
- sigma (float) – The standard deviation of the distribution for the parameter
- q (float) – The regulation parameter to bind the values with.
-
__abstractmethods__
= frozenset([])¶
-
__slotnames__
= []¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
QUniformParameter
¶
-
class
pySPACE.missions.nodes.decorators.
QUniformParameter
(parameter_name, min_value, max_value, q)[source]¶ Bases:
pySPACE.missions.nodes.decorators.UniformParameter
,pySPACE.missions.nodes.decorators.QMixin
Defines a parameter as being uniform distributed but to take only discrete values.
A uniform distributed parameter will be sampled equally distributed between a given minimum and maximum value and will be bound by a regulation parameter.
The value will be sampled from a function like this:
round(uniform(min, max) / q) * qThis parameter is bound to [floor(min), tail(max)].
>>> @QUniformParameter("test", min_value=0, max_value=10, q=0.5) ... class A(object): ... pass
Class Components Summary
__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
plot
()-
__init__
(parameter_name, min_value, max_value, q)[source]¶ Creates a new q-uniform distributed parameter with parameter_name as name.
The min_value and max_value define the borders for this distribution in between which the value will be sampled. But this distribution will be bound to discrete values by the regulation parameter q.
Parameters: - min_value (float) – The minimum value of the parameter
- max_value (float) – The maximum value of the parameter
- q (float) – The regulation parameter to bind the values with.
-
__abstractmethods__
= frozenset([])¶
-
__slotnames__
= []¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
LogNormalParameter
¶
-
class
pySPACE.missions.nodes.decorators.
LogNormalParameter
(parameter_name, shape, scale)[source]¶ Bases:
pySPACE.missions.nodes.decorators.AddedParameterDecorator
Defines a parameter as being drawn from the exponential of a normal distribution.
A log-normal distributed parameter will be sampled from exponential of the defined distribution by a mean and a standard deviation.
The values for this parameter will be sampled from a function like:
exp(normal(mu, sigma))This distribution causes that the logarithm of the samples values are being normal distributed. This parameter is bound to positive numbers only.
>>> @LogNormalParameter("test", shape=1, scale=1) ... class A(object): ... pass
Class Components Summary
__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
plot
()scale
shape
-
shape
¶
-
scale
¶
-
__abstractmethods__
= frozenset([])¶
-
__slotnames__
= []¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
LogUniformParameter
¶
-
class
pySPACE.missions.nodes.decorators.
LogUniformParameter
(parameter_name, min_value, max_value)[source]¶ Bases:
pySPACE.missions.nodes.decorators.UniformParameter
Defines a parameter as being drawn from the exponential of a uniform distribution.
A log-uniform distributed parameter will be sampled from the exponential of equally distributed values between a given minimum and maximum value.
The values for this parameter will be sampled from a function like:
exp(uniform(min, max))This distribution causes that the logarithm of the samples values are being uniform distributed. This parameter is bound to [exp(min), exp(max)].
>>> @LogUniformParameter("test", min_value=0, max_value=1000) ... class A(object): ... pass
Class Components Summary
LogUniform
(a, b)__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
plot
()-
class
LogUniform
(a, b)[source]¶ Bases:
object
-
log
= <ufunc 'log'>¶
-
__weakref__
¶ list of weak references to the object (if defined)
-
-
LogUniformParameter.
__abstractmethods__
= frozenset([])¶
-
LogUniformParameter.
__slotnames__
= []¶
-
LogUniformParameter.
_abc_cache
= <_weakrefset.WeakSet object>¶
-
LogUniformParameter.
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
LogUniformParameter.
_abc_negative_cache_version
= 33¶
-
LogUniformParameter.
_abc_registry
= <_weakrefset.WeakSet object>¶
-
class
QLogNormalParameter
¶
-
class
pySPACE.missions.nodes.decorators.
QLogNormalParameter
(parameter_name, shape, scale, q)[source]¶ Bases:
pySPACE.missions.nodes.decorators.LogNormalParameter
,pySPACE.missions.nodes.decorators.QMixin
Defines a parameter as being drawn from the exponential of a normal distribution.
A log-normal distributed parameter will be sampled from exponential of the defined distribution by a mean and a standard deviation but it will be bound to discrete values regularized by a regulation parameter.
The values for this parameter will be sampled from a function like:
round(exp(normal(mu, sigma)) / q) * qThis distribution causes that the logarithm of the samples values are being normal distributed. This parameter is bound to positive number only.
>>> @QLogNormalParameter("test", shape=1, scale=1, q=0.5) ... class A(object): ... pass
Class Components Summary
__abstractmethods__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
plot
()-
__abstractmethods__
= frozenset([])¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
QLogUniformParameter
¶
-
class
pySPACE.missions.nodes.decorators.
QLogUniformParameter
(parameter_name, min_value, max_value, q)[source]¶ Bases:
pySPACE.missions.nodes.decorators.LogUniformParameter
,pySPACE.missions.nodes.decorators.QMixin
Defines a parameter as being drawn from the exponential of a uniform distribution and being bound to discrete values only.
A q-log-uniform distributed parameter will be sampled from the exponential of equally distributed values between a given minimum and maximum value and will be bound by a regulation parameter.
The values for this parameter will be sampled from a function like:
round(exp(uniform(min, max)) / q) * qThis distribution causes that the logarithm of the samples values are being uniform distributed. This parameter is bound to [exp(min), exp(max)].
>>> @QLogUniformParameter("test", min_value=0, max_value=1000, q=0.5) ... class A(object): ... pass
Class Components Summary
__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
plot
()-
__abstractmethods__
= frozenset([])¶
-
__slotnames__
= []¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-
NoOptimizationParameter
¶
-
class
pySPACE.missions.nodes.decorators.
NoOptimizationParameter
(parameter_name)[source]¶ Bases:
pySPACE.missions.nodes.decorators.AddedParameterDecorator
Defines a previously defined parameter as not being an optimization parameter at all.
This decorator can be used in derived classes where optimization parameters of base classes are given concrete values or don’t matter at all.
>>> @NoOptimizationParameter("test") ... class A(object): ... pass
Class Components Summary
__abstractmethods__
__slotnames__
_abc_cache
_abc_negative_cache
_abc_negative_cache_version
_abc_registry
plot
()-
__abstractmethods__
= frozenset([])¶
-
__slotnames__
= []¶
-
_abc_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache
= <_weakrefset.WeakSet object>¶
-
_abc_negative_cache_version
= 33¶
-
_abc_registry
= <_weakrefset.WeakSet object>¶
-